This post shows how to implement periodic tile updates in web api with the NotificationsExtensions.Win10 NuGet package for UWP Apps.
The sample solution is here: https://github.com/gregkalapos/PeriodicLiveTileUpdateWithWebApiSample
You could do periodic updates already with Windows Store Apps in Windows 8. The cool new thing in UWP is that it comes with an Adaptive Tile Template. Meaning instead of having bunch of templates (like in Windows 8..) there is now only a single one which you can customize.
For more see this:
https://msdn.microsoft.com/en-us/library/windows/apps/xaml/mt590880.aspx
and this:
https://msdn.microsoft.com/en-us/library/windows/apps/xaml/mt186446.aspx
The other cool thing is that there is a NuGet package called NotificationsExtensions.Win10 (here it is https://www.nuget.org/packages/NotificationsExtensions.Win10/ ), which helps you to generate the xmls for the tiles in C#.
This package is not an “uwp only” package. You can also add this to the backend side, so you can generate the xmls easily on the backend side and send it to the app when it triggers an update.
In the sample solution there are two projects:
- BackendSide: It contains a Web api controller which listens to requests and generates tile xmls.
- UWPWithPeriodicLiveTile: A sample UWP app which sends tile update requests every 30minutes to the BackendSide.
Implementation of the Backend:
The first step is to add the NotificationsExtensions.Win10 package.
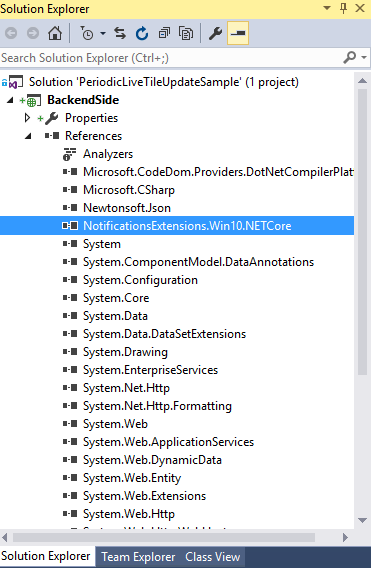
After that you can create the logic which generates the xml files with the TileContent class (it’s implemented in the NuGet package)
public class Generator
{
public static TileContent Generate()
{
Random rnd = new Random(DateTime.Now.Second);
TileContent content = new TileContent()
{ Visual = new TileVisual()
{ Branding = TileBranding.NameAndLogo,
TileSmall = new TileBinding()
Content = new TileBindingContentAdaptive()
{ Children = { new TileText()
{ Text = "PeriodicSmall" + rnd.Next(30) }
}
}
},
TileMedium = new TileBinding()
{ Content = new TileBindingContentAdaptive()
{ Children = { new TileText()
{ Text = "PeriodicMedium" + rnd.Next(30) }
}
}
}, TileWide = new TileBinding()
{ Content = new TileBindingContentAdaptive()
{ Children = { new TileText()
{ Text = "PeriodicWide" + rnd.Next(30)
} } } },
TileLarge = new TileBinding()
{ Content = new TileBindingContentAdaptive()
{ Children = { new TileText()
{ Text = "PeriodicLarge" + rnd.Next(30) }
} } } }
};
return content;
}
}
In this sample I only return the Text “Periodic[TileSize][RandomNumber]. Obviously in a real world scenario this would be something more complex.
We can use this class and the Generate method in a Web api controller:
public class TileUpdaterController : ApiController
{
public HttpResponseMessage GetNewTile()
{
var response = new HttpResponseMessage()
{
Content = new StringContent(Generator.Generate().GetContent(),
Encoding.UTF8, "application/xml")
};
return response;
}
}
One thing we have to make sure is that it returns an xml (so returning JSON would not work… the tile notifications must be XMLs)
Implementing the UWP Side
In the app you can trigger a periodic update very easily:
Windows.UI.Notifications.TileUpdateManager.
CreateTileUpdaterForApplication().
StartPeriodicUpdate(
new Uri("http://localhost:54353/api/TileUpdater/NewTile"),
Windows.UI.Notifications.PeriodicUpdateRecurrence.HalfHour);
That’s it!
You can find the sample solution here:
https://github.com/gregkalapos/PeriodicLiveTileUpdateWithWebApiSample
It contains an “almost” empty uwp app with a button. If you push the button it executes the StartPeriodicUpdate method and starts to query the web api for tile updates. Before you click on it make sure you pin the tile onto the start screen! ;) You should see the first update immediately.